Refs
- Cheap 1 year US Programs
- US VS Can
- Can Free Run
- Running Programs
- Manitoba Analysis
- Manitoba and NB
- Graduate Diploma for family benefits
- Points of notice
US Solution
Programs
University |
Program |
Span |
Application time |
Requirements |
Tuition |
Features |
UCI |
MCS |
15 months |
2022.10.3~2023.3.1 |
TOEFL 80, IELTS 7(each >= 6), 3 rec letters |
around 50k |
|
SJSU |
|
|
|
|
|
Bay area |
USC |
|
|
|
|
|
Los Angelos |
Santa Clara |
|
|
|
|
|
Bay area |
USF |
|
|
|
|
|
San Francisco, Close to F, G |
SFSU |
|
|
|
|
|
San Francisco, Close to F, G |
UW-Tacoma |
|
|
|
|
|
Seattle |
Northeastern University Seattle |
|
|
|
|
|
Seattle |
NYU Tandon |
|
|
|
|
|
NY |
US Visa
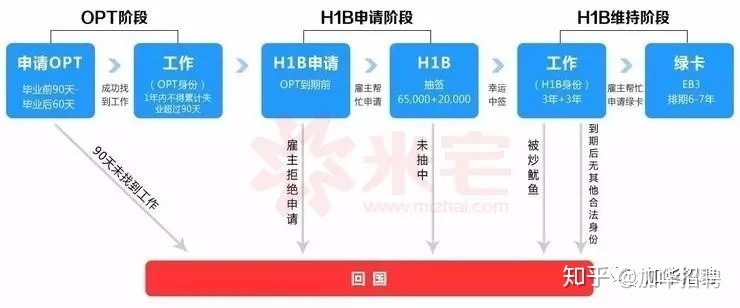
Canada Solution
BCPNP
Check out BC PNP programs!
NEU Vancouver:
- MSCS
- 32 credits, 32×1450=46400
- IELTS 7.5/TOEFL 100/Duolingo 125, GRE optional
- tried to submit 2022.11.30, failed due to technical issues
- submitted 12.1
FDU Vancouver:
- MSACS
- 2.75, GRE, 3 rec
- PR after graduation!
- paid not submitted, requires GRE and 3 rec, some said waived with a master degree(chinese degree eligible)
- Needs last rec
- offer 2023.2.14
NYIT
- Only cybersecurity program provided
- One year!
- submitted 2022.11.14, check out
- offer 2023.2.15
SFU
- Programs
- Computer Science MSc(Course, Project, Thesis)
- Master of Science in Professional Computer Science(Course, Project)
- Big Data MSc(Not found)
- Computing Science Dual Degree MSc(Thesis, not recommended)
- Computing Science Non-Thesis MSc(Not found)
- 3.0/4.33, 3/4(china), TOEFL 93(20 each), IELTS 7(6.5 each)
- Apply
- Needs IELTS and 3 recs
- Needs last rec to submit
- submitted 2023.1.19, may missed the deadline
TRU
UBC
- App
- Okanagan Programs
- Master of Data Science
- Master of Science in Computer Science
- Master of Science in Computer Science in Interdisciplinary Studies
- Vancouver Programs
- Master of Data Science
- Master of Software Systems
- Master of Science in Bioinformatics
- Master of Science in Computer Science
- Master of Science in Computer Science Sub-Specialization in HumanComputer Interaction
- Master of Applied Science in Electrical & Computer Engineering
- Not accepting Duolingo, suspended.
UNBC
UVic
- Programs
- MSCS
- Master of Applied Science in Computer Engineering
- Master of Engineering in Computer Engineering
- Master of Applied Science in Electrical and Computer Engineering
- Master of Engineering in Electrical and Computer Engineering
- Master of Engineering in Applied Data Science
- Master of Science in Health Informatics
- Low ranking, easier maybe
- MEng in Applied Data Science is 1 year and no thesis!
- Apply at official site
- Apply at EducationPlannerBC
- Duolingo not eligible, suspended.
- two assessment reports, waiting.
- Paid, waiting
OINP
此处主要分析OINP的Masters Graduate Stream项目。
核心要求
- 必须受邀(受邀机制见OINP invitations to apply)
- 教育程度
- 硕士学位
- 至少一年课程
- 在认可的大学列表中(2023年1月):Algoma University, Brescia University College (Affiliated with the University of Western Ontario), Brock University, Carleton University, Dominican University College, Huron University College (Affiliated with the University of Western Ontario), King’s University College at the University of Western Ontario, Lakehead University, Laurentian University, McMaster University, Nipissing University, Ontario College of Art & Design University, Queen’s University, Royal Military College of Canada, Ryerson University, Saint Paul University (Federated with the University of Ottawa), St. Jerome’s University (Federated with the University of Waterloo), Trent University, University of Guelph, University of Ontario Institute of Technology, University of Ottawa, University of St. Michael’s College (Federated with the University of Toronto), University of Toronto, University of Trinity College (Federated with the University of Toronto), University of Waterloo, University of Windsor, Victoria University (Federated with the University of Toronto), Western University, Wilfrid Laurier University, York University
- 语言:英语或者法语至少达到CLB7。CLB对照表
- CLB7: 6/6/6/6 CLB8: 7.5/6.5/6.5/6.5 CLB9: 8/7/7/7 CLB10: 8.5/8/7.5/7.5
Ontario EOI
Ontario Expression of interest
The EOI is comprised of two components, for a maximum EOI score of 200 points:
- self-declared score (maximum 126 points)
- business concept score (maximum 74 points)
OINP will assess and score your business concept. Your business concept will need to score at least 37 of the available 74 points (50%) to be placed in the EOI selection pool.
Whether you are issued an invitation to apply (ITA) is based on your EOI rank in the selection pool. Only the top ranked candidates are invited to apply.
打分
- Study/Work Permit: 10分
- 收入:40k以上3分
- STEM:12分
- 教育经历:加拿大本地2年以上本一年以上硕:一个5分两个10分
- 语言:
- 官方语言(英法):一门5分
- CLB7-4分 CLB8-6分 CLB9/10-10分
- 地域:
- Northern Ontario – 10 points
- Other areas outside GTA (except Northern Ontario) – 8 points
- Inside GTA (except Toronto) – 3 points
- Toronto – 0 points
申报
- Western University
Others
Graduate diploma is cheap(cheaper than master), quick(often one year) and has low bars(lower than master).
Great for family. Spouse can get a work visa and kids can receive free compulsory education.
Even if not qualified for CS master, apply other majors like engineering, mechanics, auto repair, electrician can also get a job easily.
Manitoba is ideal for graduate diploma. One year diploma, get a job and IELTS 6/6/6/6 to apply. Said to be the easiest.
NB is even easier. Same as manitoba and can apply before graduation if got work experience.
UWinnipeg (Graduate diploma)
Network Security
Web Development
Artificial Intelligence
UManitoba (Graduate diploma)
- Computer Science 2 years too long
- No satisfying extended education program, gave up.
Red River Polytech (College)
University of Regina (Master)
Concordia University
Visa
- 1 year program gives 1 year work permit, 2 year program gives 3 year work permit
WES
Ref
- 哪些大学需要WES?
- 没忍住,我把WES学历认证曝光了..
概念
- World Education Services,帮助想在美国或是加拿大留学的学生提供本科成绩认证及学历认证。简单来说是为了统一国际生的GPA算法标准。
分类
- WES 提供的评估服务分为两种:WES ICAP和WES Basic。这两种又细分为Course-by-Course; Document-by-Document以及CPA Board Evaluation。
- ICAP和Basic的区别在于,ICAP可以给申请者在WES系统里存档,以后需要WES报告的时候,WES会直接查看系统然后发报告给收件人。而Basic则是做完这一次报告之后WES系统里就不再有申请者的信息了。
- 一般我们都选择ICAP,因为系统存档后,之后需要再寄送认证至申请学校时,直接在官网order就可以了。
计分调整
(1)核心基础课-权重较高
商科主要指的数学课,比如高数、微积分、线代、概率论这些课程,专业课程比如宏微观经济学,这些课程的学分一般都很高,认证的时候权重也很高。
(2)大陆特色课-权重降低/变0
大学英语从5分降为2分,毛邓理论从4分降为1分,毕业论文从16分降为4分。体育、军事理论、认识实习、思想政治课社会实践、大学生职业规划就业指导、形式与政策等涉及到体育类,政策类,社会实践和指导类课程均为0学分。
(3)选修课-维持/降学分
专业选修课比较重要,一般维持原有学分;公共选修课会被降学分或者按0学分处理。
申请ICAP Course by Course
- 选择 Standard Application->Graduate Admissions->添加学历(只认证最高学历)->
Others
- RNIP Vernon
Glossary
coop
Align: The Northeastern University Align program provides a direct path to a Master of Science in Computer Science (MSCS) for non-computer science majors without programming experience.